Javascript Canvas User Upload Image Without Html
Image resizing is computationally expensive and usually done on the server-side so that right-sized image files are delivered to the client-side. This approach too saves data while transmitting images from the server to the client.
However, at that place are a couple of situations where you might need to resize images purely using JavaScript on the client side. For example -
- Resizing images before uploading to server
Uploading a large file on your server will take a lot of fourth dimension. You tin first resize images on the browser and then upload them to reduce upload time and better application performance.
- Rich image editors that work on client-side
A rich image editor that offers image resize, crop, rotation, zoom IN and zoom OUT capabilities often require image manipulation on the client-side. The speed is critical for the user in these editors.
If a user is manipulating a heavy paradigm, it volition have a lot of time to download transformed images from the server. Imagine this with operations like undo/redo and circuitous text and image overlays.
Image manipulation in JavaScript is done using the canvas chemical element. In that location are libraries like textile.js that offer rich APIs.
Apart from the above two reasons, in almost all cases, y'all would desire to become the resized images from the backend itself then that client doesn't accept to deal with heavy processing tasks.
In this post-
- We will kickoff talk near how to do resizing purely in JavaScript using the
canvas
chemical element.
- Then we will embrace in great particular how yous tin can resize, crop, and exercise a lot with images by changing the epitome URL in the
src
attribute. This is the preferred manner to resize images without degrading the user feel programmatically.Also, we will learn how yous can do this without needing to set any libraries or backend servers.
Paradigm resizing in JavaScript - Using canvas element
The HTML <canvas>
element is used to depict graphics, on the fly, via JavaScript. Resizing images in browser using canvas
is relatively elementary.
drawImage
function allows usa to return and scale images on canvas element.
drawImage(paradigm, x, y, width, meridian)
The first argument image
tin be created using the Image()
constructor, as well as using any existing <img>
element.
Allow'southward write the lawmaking to resize a user-uploaded image on the browser side 300x300
.
<html> <body> <div> <input type="file" id="image-input" take="image/*"> <img id="preview"></img> </div> <script> let imgInput = certificate.getElementById('paradigm-input'); imgInput.addEventListener('change', function (e) { if (e.target.files) { let imageFile = e.target.files[0]; var reader = new FileReader(); reader.onload = function (east) { var img = document.createElement("img"); img.onload = function (event) { // Dynamically create a canvas element var canvas = certificate.createElement("canvas"); // var canvas = document.getElementById("canvas"); var ctx = canvas.getContext("2d"); // Actual resizing ctx.drawImage(img, 0, 0, 300, 300); // Bear witness resized image in preview chemical element var dataurl = canvas.toDataURL(imageFile.type); certificate.getElementById("preview").src = dataurl; } img.src = e.target.result; } reader.readAsDataURL(imageFile); } }); </script> </body> </html>
Permit's sympathize this in parts. First, the input file type field in HTML
<html> <body> <div> <input type="file" id="epitome-input" have = "image/*"> <img id="preview"></img> </div> </body> </html>
Now we demand to read the uploaded image and create an img
element using Prototype()
constructor.
let imgInput = certificate.getElementById('image-input'); imgInput.addEventListener('change', part (east) { if (e.target.files) { let imageFile = east.target.files[0]; var reader = new FileReader(); reader.onload = part (e) { var img = document.createElement("img"); img.onload = function(issue) { // Bodily resizing } img.src = e.target.result; } reader.readAsDataURL(imageFile); } });
Finally, let'southward draw the image on sail and prove preview chemical element.
// Dynamically create a canvass chemical element var canvas = document.createElement("canvas"); var ctx = canvas.getContext("second"); // Actual resizing ctx.drawImage(img, 0, 0, 300, 300); // Show resized prototype in preview element var dataurl = canvas.toDataURL(imageFile.type); document.getElementById("preview").src = dataurl;
You might notice that the resized image looks distorted in a few cases. It is because we are forced 300x300
dimensions. Instead, we should ideally only manipulate one dimension, i.eastward., acme or width, and conform the other accordingly.
All this tin can be done in JavaScript, since you have access to input image original height (img.width
) and width using (img.width
).
For example, we can fit the output image in a container of 300x300
dimension.
var MAX_WIDTH = 300; var MAX_HEIGHT = 300; var width = img.width; var height = img.top; // Change the resizing logic if (width > pinnacle) { if (width > MAX_WIDTH) { height = height * (MAX_WIDTH / width); width = MAX_WIDTH; } } else { if (height > MAX_HEIGHT) { width = width * (MAX_HEIGHT / height); tiptop = MAX_HEIGHT; } } var canvass = document.createElement("canvas"); canvas.width = width; canvas.meridian = superlative; var ctx = canvas.getContext("second"); ctx.drawImage(img, 0, 0, width, summit);
Decision-making image scaling behavior
Scaling images tin can consequence in fuzzy or blocky artifacts. There is a merchandise-off between speed and quality. Past default browsers are tuned for better speed and provides minimum configuration options.
You tin play with the following properties to control smoothing issue:
ctx.mozImageSmoothingEnabled = false; ctx.webkitImageSmoothingEnabled = false; ctx.msImageSmoothingEnabled = false; ctx.imageSmoothingEnabled = false;
Epitome resizing in JavaScript - The serverless mode
ImageKit allows you to manipulate image dimensions directly from the image URL and get the verbal size or crop you lot want in real-fourth dimension. Start with a unmarried primary prototype, every bit large equally possible, and create multiple variants from the same.
For example, nosotros tin create a 400 ten 300
variant from the original epitome like this:
https://ik.imagekit.io/ikmedia/ik_ecom/shoe.jpeg?tr=w-400,h-300
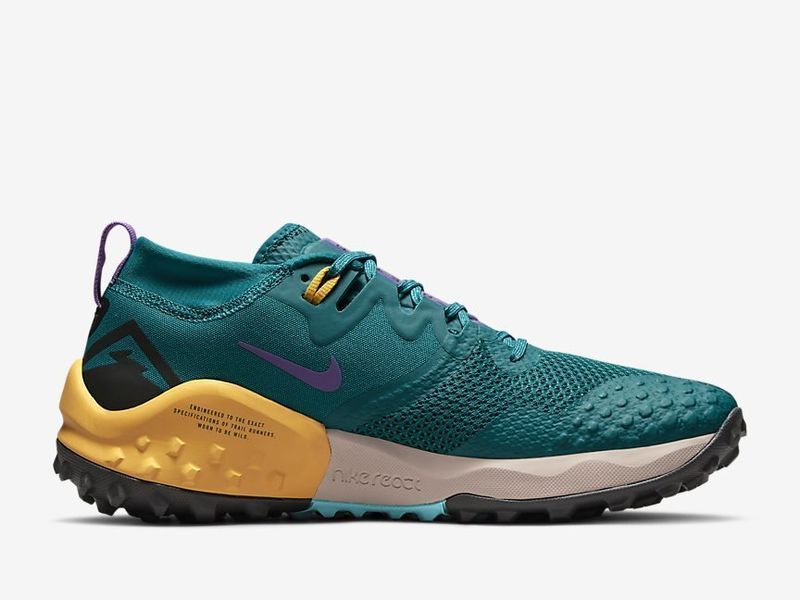
You can use this URL direct on your website or app for the product image, and your users go the correct image instantly.
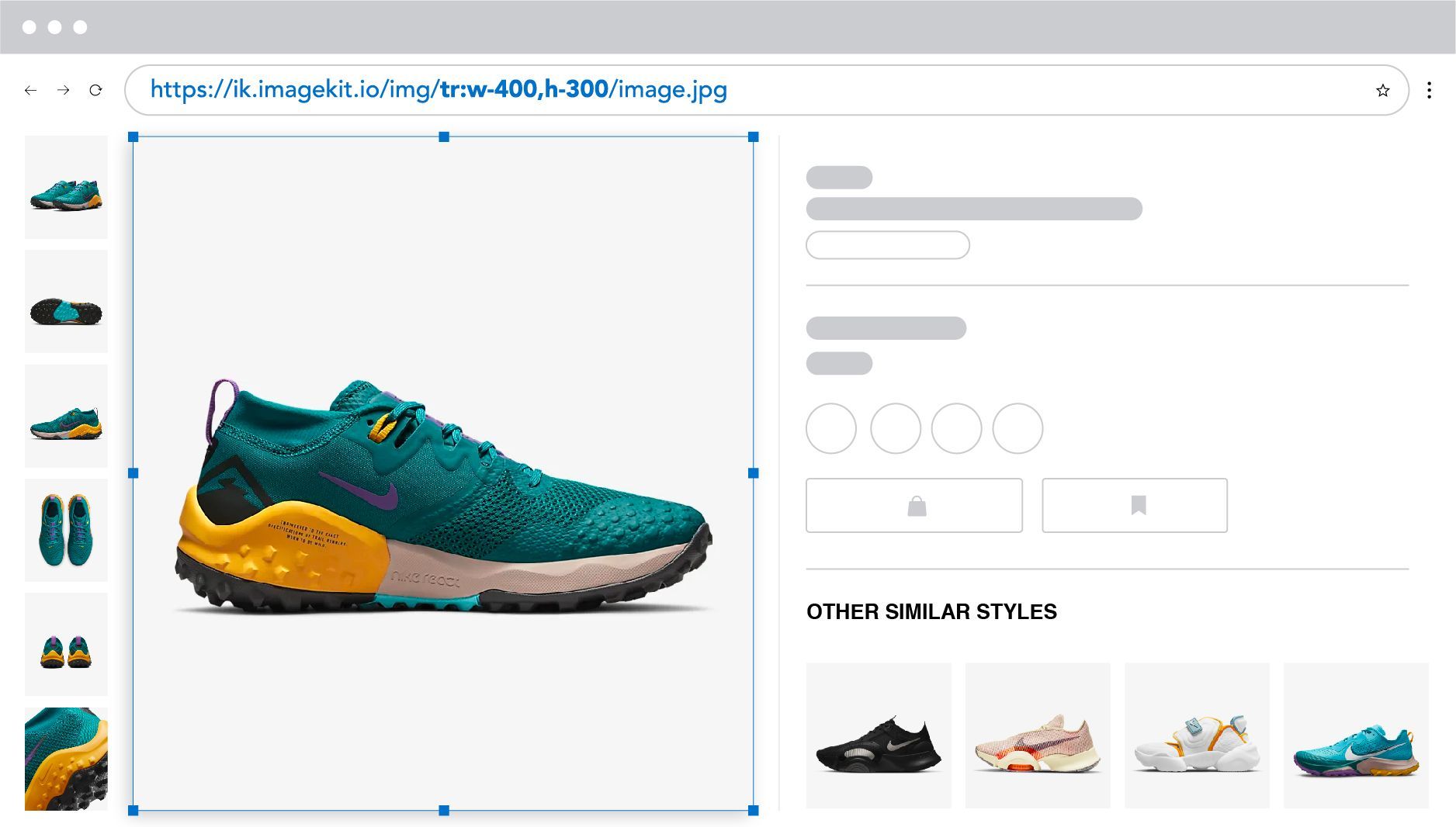
If you don't want to crop the image while resizing, in that location are several possible crop modes.
https://ik.imagekit.io/ikmedia/ik_ecom/shoe.jpeg?tr=w-400,h-300,cm-pad_resize,bg-F5F5F5
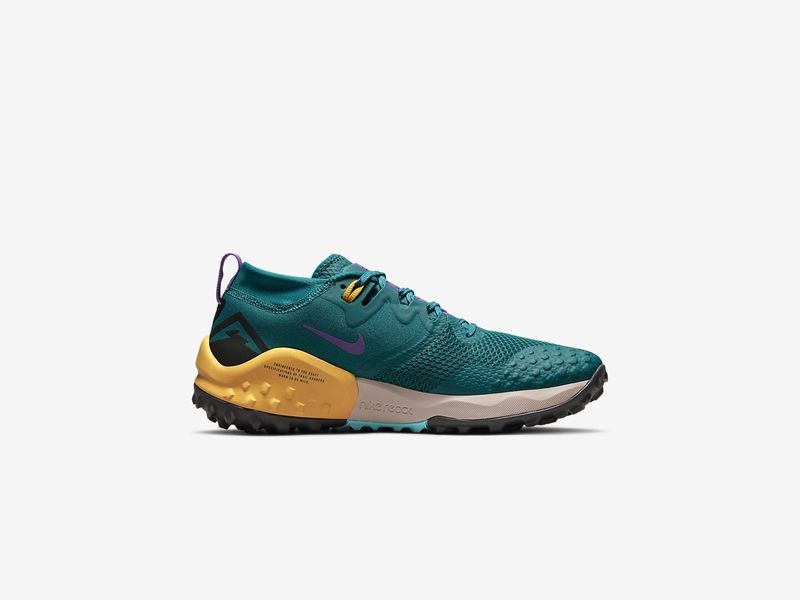
Nosotros accept published guides on how you can exercise the following things using ImageKit's real-time paradigm manipulation.
- Resize image - Basic elevation & width manipulation
- Cropping & preserving the aspect ratio
- Face and object detection
- Add a watermark
- Add together a text overlay
- Adapt for slow net connection
- Loading a blurred low-quality placeholder
Summary
- In most cases, y'all should non do prototype resizing in the browser because it is tedious and results in poor quality. Instead, y'all should use an image CDN like ImageKit.io to resize images dynamically by changing the image URL. Try our forever free program today!
- If your use-instance demands customer-side resizing, it is possible using the
canvas
element.
Source: https://imagekit.io/blog/how-to-resize-image-in-javascript/
0 Response to "Javascript Canvas User Upload Image Without Html"
Post a Comment